Ever wondered how information travels between different parts of your JavaScript code? Well, that’s all about how data is passed around! In this post, we’ll explore two ways this happens: pass by value and pass by reference. These terms might sound fancy, but they’re actually quite easy to understand.
Imagine your computer’s memory as a big storage room. When you create a variable in JavaScript, it’s like reserving a tiny box in this room. This box holds the value you give to the variable.
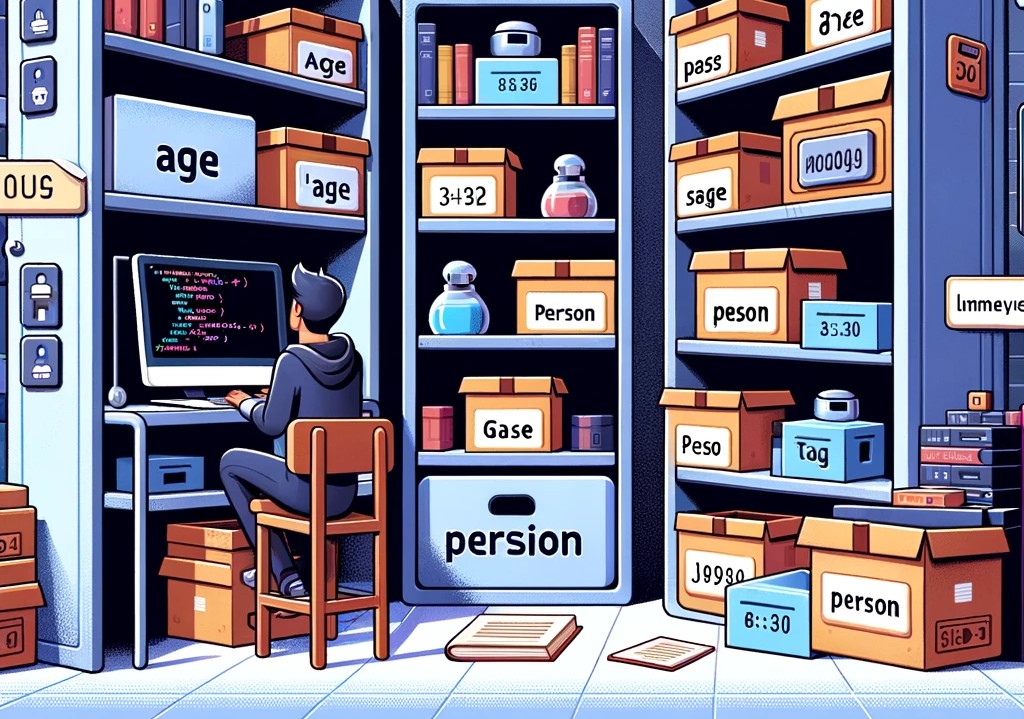
For instance:
let age = 30;
Here, we create a box named age
and put the number 30 inside. Think of age
as a label stuck on the box.
Pass by Value: Making Copies
JavaScript mostly uses a concept called “pass by value” for basic things like numbers and words. Here’s the idea:
- When you give a value to a function, it gets a copy of that value.
- The function can change this copy, but the original value in your code stays the same.
Imagine borrowing a cup of sugar from your neighbor. You get a copy (the borrowed sugar), and your neighbor’s supply (the original value) stays untouched.
Here’s an example:
function changeAge(currentAge) {
currentAge = currentAge + 10; // Modify the copy
}
let myAge = 25;
changeAge(myAge);
console.log(myAge); // Output: 25 (Original value stays unchanged)
See how changeAge
receives a copy of myAge
(25) and adds 10 to it. This change doesn’t affect the original value of myAge
outside the function.
Pass by Reference: Sharing Locations
Things work a little differently with more complex things like lists and objects in JavaScript. Here, JavaScript uses “pass by reference.” Let’s break it down:
- When you give an object to a function, JavaScript doesn’t copy the entire object. Instead, it gives the function a special note (like a room number) that tells it where the object is stored in memory.
- Any changes made to the object’s details within the function will affect the original object because both the function and the original variable have the same “room number” for the object.
Imagine borrowing a book from the library. You’re not given a copy of the book; you get the book itself (the object). Any notes you take (modifications) will be there when someone else borrows the same book (the original object is affected).
Here’s an example:
function updatePerson(person) {
person.name = "Alice"; // Modify the property of the original object
}
let person = { name: "Bob" };
updatePerson(person);
console.log(person.name); // Output: "Alice" (Original object is modified)
In this example, updatePerson
receives a “room number” for the person
object. When it changes the name
property, it modifies the original object itself, as both the function and the original variable point to the same location in memory.
Remember This!
Understanding pass by value and pass by reference is important for writing clear and predictable JavaScript code. Here’s a quick tip:
- Use pass by value for basic things like numbers and words when you don’t want the function to change the original value.
- Use pass by reference for more complex things like lists and objects when you intend to modify the object’s details within the function.
By mastering these concepts, you’ll be well on your way to writing awesome JavaScript code!